How To Loop Through an Array with PHP
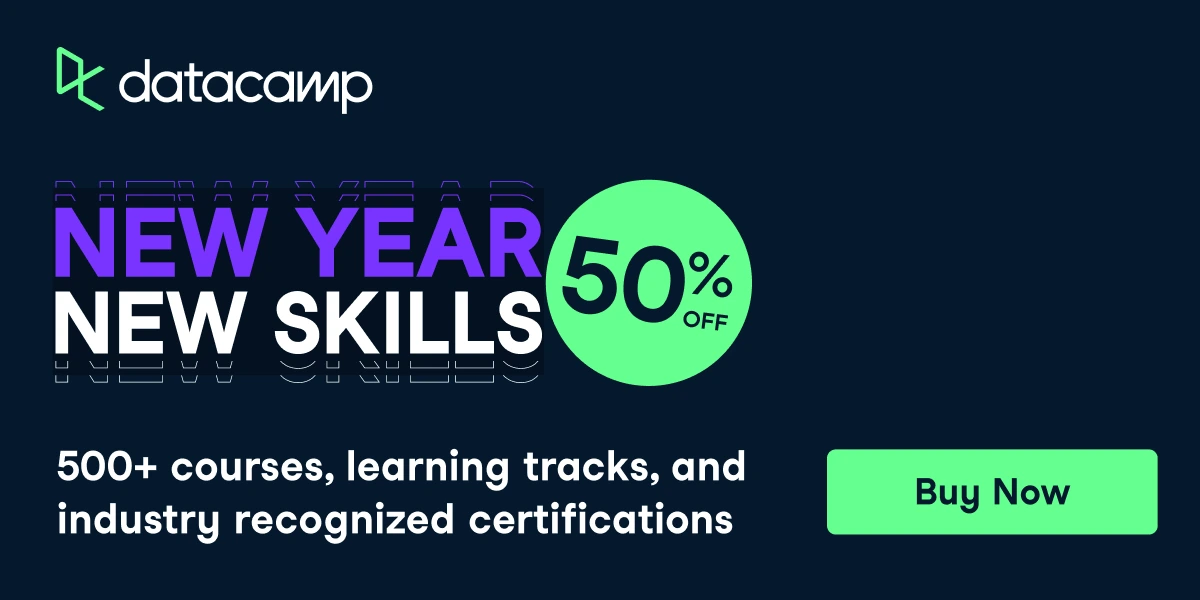
* Web 2.0 University is supported by it's audience. If you purchase through links on our site, we may earn an affiliate commision.
Looping through arrays is a fundamental skill for PHP developers. Whether you’re working with simple lists or complex data structures, understanding various methods to iterate through arrays can significantly improve your code’s efficiency and readability. In this post, we’ll explore different ways to loop through arrays in PHP, including built-in functions, custom approaches, and tips for choosing the most appropriate method for your needs.
1. The Classic: foreach Loop
The foreach
loop is the most common and straightforward way to iterate through an array in PHP. It’s versatile and works well for both indexed and associative arrays.
$fruits = ['apple', 'banana', 'cherry'];
foreach ($fruits as $fruit) {
echo $fruit . "\n";
}
For associative arrays, you can easily access both keys and values:
$person = ['name' => 'John', 'age' => 30, 'city' => 'New York'];
foreach ($person as $key => $value) {
echo "$key: $value\n";
}
2. The Traditional: for Loop
When working with indexed arrays and you need more control over the iteration process, the for
loop can be useful:
$numbers = [1, 2, 3, 4, 5];
$count = count($numbers);
for ($i = 0; $i < $count; $i++) {
echo $numbers[$i] . "\n";
}
3. Built-in Functions: array_map() and array_walk()
PHP offers built-in functions that can be more concise for certain operations:
array_map()
applies a callback function to each element of an array:
$numbers = [1, 2, 3, 4, 5];
$squared = array_map(function($n) { return $n * $n; }, $numbers);
array_walk()
is similar but can modify the original array and work with keys:
$fruits = ['a' => 'apple', 'b' => 'banana', 'c' => 'cherry'];
array_walk($fruits, function(&$value, $key) {
$value = strtoupper($value);
});
4. The Quick and Dirty: while and each()
While not recommended for new code due to potential performance issues, the each()
function with a while
loop can be found in legacy code:
$person = ['name' => 'John', 'age' => 30, 'city' => 'New York'];
while ($data = each($person)) {
echo $data['key'] . ': ' . $data['value'] . "\n";
}
5. Custom Functions for Special Cases
Sometimes, you might need a custom function for specific requirements. Here’s an example of a recursive function to loop through a multi-dimensional array:
function arrayLoop($array) {
foreach ($array as $key => $value) {
if (is_array($value)) {
arrayLoop($value);
} else {
echo "$key: $value\n";
}
}
}
$nestedArray = [
'fruits' => ['apple', 'banana'],
'person' => ['name' => 'John', 'age' => 30]
];
arrayLoop($nestedArray);
Choosing the Right Method
When deciding which method to use, consider these factors:
- Readability:
foreach
is often the most readable for simple iterations. - Performance: For large arrays,
for
loops can be slightly faster. - Functionality: Built-in functions like
array_map()
can be more concise for specific operations. - Array Type: Some methods work better with indexed arrays, others with associative arrays.
Conclusion
Mastering different ways to loop through arrays in PHP can make your code more efficient and expressive. While foreach
is often the go-to method for its simplicity and versatility, understanding alternatives like for
loops, built-in functions, and custom approaches allows you to choose the best tool for each specific task. Remember, the most appropriate method depends on your specific use case, the type of array you’re working with, and the operations you need to perform.
By leveraging these techniques, you can write cleaner, more efficient PHP code when working with arrays.