PHP cURL With Headers
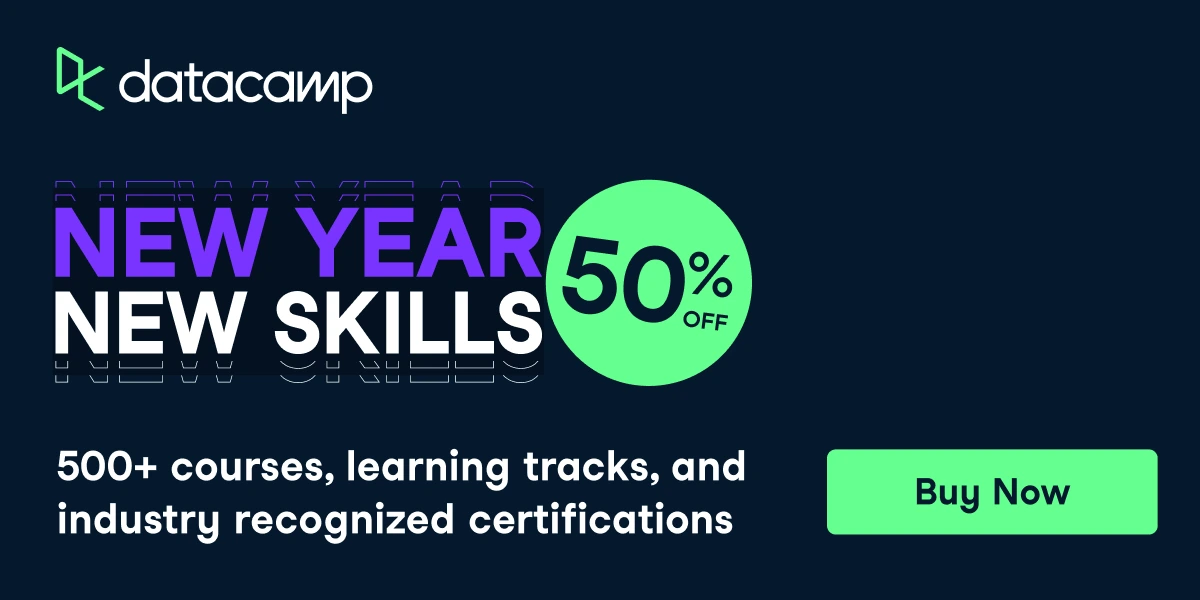
* Web 2.0 University is supported by it's audience. If you purchase through links on our site, we may earn an affiliate commision.
TL;DR
The key to sending PHP cURL requests with headers is to set the CURLOPT_HTTPHEADER
option with the curl_setopt
function like so:
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
Read on for some more detailed examples.
Setting Up a Basic cURL Request with Headers
Let’s start with the basics of sending a cURL request with custom headers:
<?php
// Initialize cURL session
$ch = curl_init();
// Set the URL
curl_setopt($ch, CURLOPT_URL, "https://api.example.com/endpoint");
// Set custom headers
$headers = array(
"Content-Type: application/json",
"Authorization: Bearer YOUR_ACCESS_TOKEN"
);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
// Execute the request and close cURL session
$response = curl_exec($ch);
curl_close($ch);
// Process the response
echo $response;
?>
In this example, we’re setting two common headers: Content-Type
and Authorization
. The CURLOPT_HTTPHEADER
option allows us to pass an array of headers to be sent with the request.
Types of Headers
There are various types of headers you can use with PHP cURL. Here are some common categories:
Request Headers: Sent by the client to provide additional information about the request.
User-Agent
: Identifies the client software.Accept
: Specifies which content types are acceptable for the response.Authorization
: Provides authentication credentials.
Response Headers: Sent by the server to provide additional information about the response.
Content-Type
: Indicates the media type of the resource.Cache-Control
: Specifies caching directives.Set-Cookie
: Sets a cookie at the client.
Representation Headers: Describe the particular representation of the resource sent in the message body.
Content-Type
: Indicates the media type of the resource.Content-Encoding
: Specifies any encodings applied to the payload.
Payload Headers: Contain information about the payload of the message.
Content-Length
: Indicates the size of the payload body.Content-Range
: Indicates where in the full resource a partial message belongs.
Practical Examples
Let’s look at some typical scenarios where you might use headers with PHP cURL:
1. Sending JSON Data
<?php
$ch = curl_init("https://api.example.com/users");
$data = json_encode(array("name" => "John Doe", "email" => "[email protected]"));
curl_setopt($ch, CURLOPT_POSTFIELDS, $data);
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Content-Type: application/json',
'Content-Length: ' . strlen($data)
));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
This example sends a POST request with JSON data, setting the appropriate Content-Type
and Content-Length
headers.
2. Basic Authentication
<?php
$ch = curl_init("https://api.example.com/protected-resource");
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'Authorization: Basic ' . base64_encode("username:password")
));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
This example demonstrates how to use Basic Authentication by setting the Authorization
header.
3. Working with Cookies
<?php
$ch = curl_init("https://api.example.com/login");
curl_setopt($ch, CURLOPT_COOKIEJAR, 'cookies.txt');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
// Subsequent request using the saved cookies
$ch = curl_init("https://api.example.com/profile");
curl_setopt($ch, CURLOPT_COOKIEFILE, 'cookies.txt');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
curl_close($ch);
echo $response;
?>
This example shows how to handle cookies across multiple requests, which is useful for maintaining session state.
Conclusion
Understanding how to work with headers in PHP cURL opens up a world of possibilities for interacting with web services and APIs. From authentication to content negotiation, headers play a vital role in HTTP communication. By mastering these techniques, you’ll be well-equipped to handle a wide range of web development scenarios.
Remember to always refer to the API documentation of the service you’re working with, as different APIs may require specific headers or have unique requirements.