How to Get an Exception Message in PHP
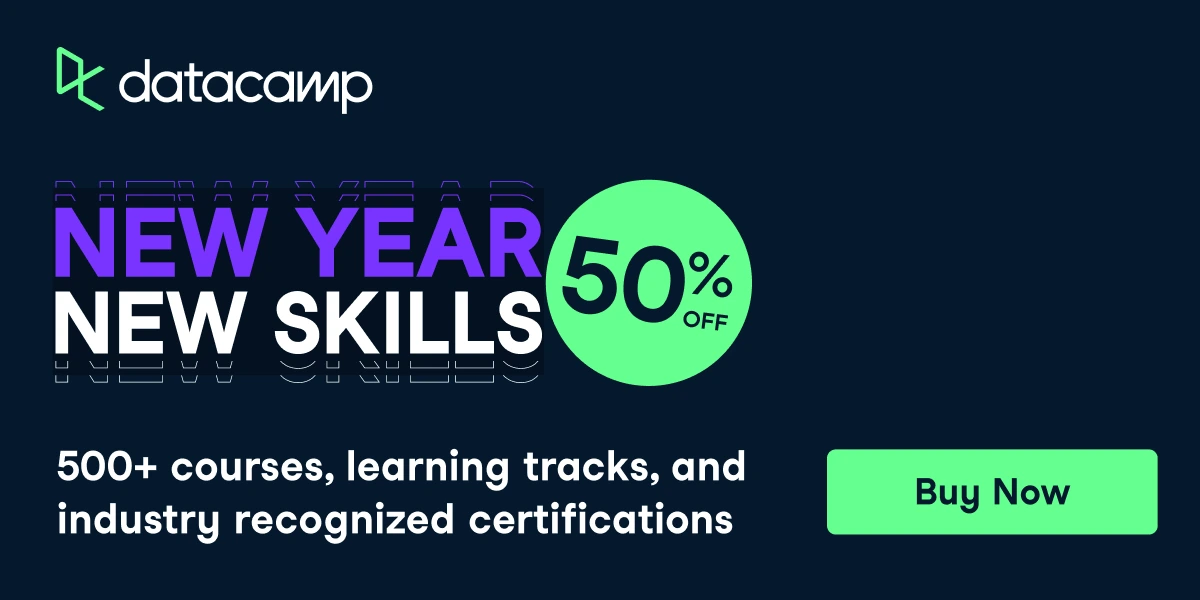
* Web 2.0 University is supported by it's audience. If you purchase through links on our site, we may earn an affiliate commision.
Exception handling is a key feature in PHP that allows developers to manage and respond to errors in a controlled way. When an exception is thrown, usually a specific exception message has been set and it carries an important message that can provide details about what went wrong.
PHP offers a convenient way to retrieve this message through the getMessage()
method. In this blog post, we’ll explore how to get an exception message in PHP, its significance, and go through examples of how it works in real-world scenarios.
How to Get an Exception Message in PHP
In PHP, exceptions are represented by instances of the Exception
class. When an exception is thrown, a message can be included to describe the error. To retrieve this message, you can use the getMessage()
method of the exception object. This is particularly useful for debugging and logging errors, as it provides insights into the cause of the issue.
Syntax
Here’s the basic syntax for retrieving an exception message:
try {
// Code that may throw an exception
} catch (Exception $e) {
echo $e->getMessage();
}
getMessage()
: This method retrieves the message that was passed to the exception when it was thrown.
Key Points
- The
getMessage()
method is part of the baseException
class and can be used in any custom exception classes that extend it. - The message helps in identifying the error source and is often combined with other exception methods like
getCode()
orgetFile()
to provide more details. - It is best practice to set informative exception messages to help track down problems in your code.
Examples of Using getMessage()
in PHP
Now, let’s dive into some practical examples of using the getMessage()
method in various scenarios.
Example 1: Basic Exception Handling with getMessage()
In this example, we will throw a simple exception and retrieve its message using the getMessage()
method:
try {
throw new Exception("This is a custom error message.");
} catch (Exception $e) {
echo "Error: " . $e->getMessage();
}
Output:
Error: This is a custom error message.
In this case, an exception is thrown with a custom error message, and the catch
block captures it. The getMessage()
method then retrieves the error message, which is displayed to the user.
Example 2: Handling Division by Zero
A common scenario where exceptions are useful is handling division by zero errors. Let’s look at an example:
function divide($a, $b) {
if ($b == 0) {
throw new Exception("Division by zero is not allowed.");
}
return $a / $b;
}
try {
echo divide(10, 0);
} catch (Exception $e) {
echo "Caught exception: " . $e->getMessage();
}
Output:
Caught exception: Division by zero is not allowed.
In this example, we’ve created a function that throws an exception when trying to divide by zero. The catch
block then retrieves the exception message using getMessage()
, and displays it.
Example 3: Using Custom Exception Classes
While you can always use the built-in Exception
class, you might want to create your own custom exception classes to handle specific error conditions more gracefully. Here’s an example of how to retrieve an exception message from a custom exception:
class InvalidInputException extends Exception {}
function checkInput($input) {
if ($input < 0) {
throw new InvalidInputException("Input cannot be negative: $input");
}
return true;
}
try {
checkInput(-10);
} catch (InvalidInputException $e) {
echo "Custom Exception Caught: " . $e->getMessage();
}
Output:
Custom Exception Caught: Input cannot be negative: -10
In this case, a custom exception InvalidInputException
is thrown when the input is negative. The message includes dynamic information about the invalid input value, and the getMessage()
method retrieves this specific message.
Example 4: Combining getMessage()
with Other Exception Methods
In many cases, you might want to display more than just the exception message. PHP’s Exception
class provides other methods, like getCode()
, getFile()
, and getLine()
, which can offer additional context when an error occurs.
try {
throw new Exception("A critical error occurred", 404);
} catch (Exception $e) {
echo "Error: " . $e->getMessage() . " in " . $e->getFile() . " on line " . $e->getLine() . " (Code: " . $e->getCode() . ")";
}
Output:
Error: A critical error occurred in /path/to/your/script.php on line 3 (Code: 404)
Here, we’re using multiple exception methods (getMessage()
, getFile()
, getLine()
, and getCode()
) to give a more complete description of the error, which can be helpful for debugging or logging purposes.
Why Getting Exception Messages is Important
Proper error handling, including retrieving and using exception messages, is a crucial part of maintaining reliable applications. Here are some reasons why using getMessage()
to retrieve exception messages is important:
- Clear Debugging Information: The message helps developers understand the specific issue that occurred, making it easier to debug and fix.
- User-Friendly Error Reporting: You can show more meaningful messages to users, allowing them to understand what went wrong without revealing sensitive system details.
- Better Logging: When logging errors in production environments, capturing and saving exception messages helps in diagnosing issues later.
- Graceful Error Handling: By catching exceptions and accessing their messages, you can avoid crashes and provide a smoother experience for users.
Conclusion
Retrieving exception messages using the getMessage()
method is an essential part of PHP’s exception handling mechanism. It allows developers to handle errors more effectively by providing informative and specific error messages. Whether you’re working with built-in or custom exceptions, understanding how to get and use exception messages is crucial for improving the maintainability and robustness of your applications.
By incorporating exception messages into your error handling process, you’ll be able to quickly pinpoint issues and ensure that your code handles errors gracefully.
Additional Resources:
With these insights and examples, you’ll have a better understanding of how to effectively retrieve and use exception messages in your PHP applications.