How to Upload a File to a Server from a URL Using PHP
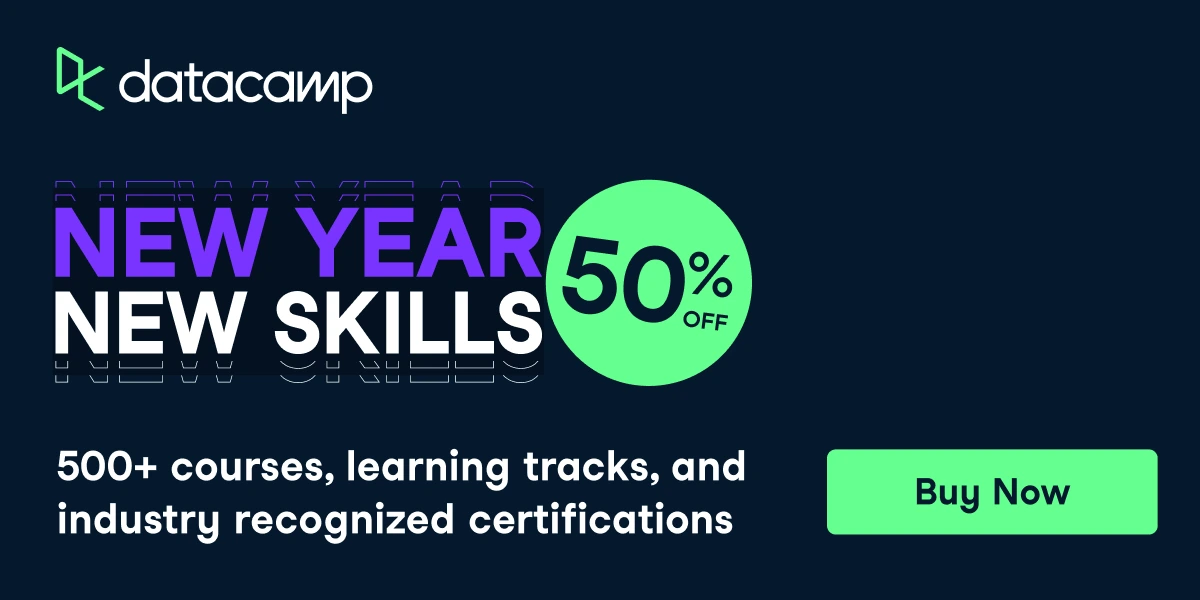
* Web 2.0 University is supported by it's audience. If you purchase through links on our site, we may earn an affiliate commision.
Uploading files from a URL directly to a server is a useful feature for web applications, especially when automating content fetching or integrating external resources.
This tutorial will show you how to use PHP to handle file uploads from URLs, including addressing challenges like redirects, HTTP errors, and authorization.
Outline
- Introduction
- Use Cases for Uploading Files from a URL
- Step-by-Step Guide
- Basic PHP Code for File Upload from URL
- Handling Redirects and HTTP Errors
- Managing Authorization for Restricted URLs
- Conclusion
- Additional Resources
Introduction
Uploading a file to a server from a URL involves fetching a file hosted on an external server and saving it to your server. This approach eliminates the need for users to download and re-upload files manually. Let’s explore how to handle a PHP file upload from a URL, ensuring smooth functionality even when dealing with redirects, 404 errors, or authentication.
Use Cases for Uploading Files from a URL
- Content Aggregation: Automating downloads of images, videos, or documents from external sources.
- Data Backup: Transferring resources between servers for storage or backup purposes.
- API Integrations: Fetching files from third-party services that provide public or authenticated URLs.
Step-by-Step Guide
1. Basic PHP Code for File Upload from URL
The following example demonstrates a simple implementation:
<?php
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$url = $_POST['file_url'];
// Validate the URL
if (!filter_var($url, FILTER_VALIDATE_URL)) {
die("Invalid URL provided.");
}
// Fetch file contents from the URL
$fileContents = file_get_contents($url);
if ($fileContents === false) {
die("Failed to fetch the file from the URL.");
}
// Determine file name and save it
$fileName = basename(parse_url($url, PHP_URL_PATH));
$savePath = 'uploads/' . $fileName;
if (file_put_contents($savePath, $fileContents)) {
echo "File uploaded successfully to $savePath";
} else {
echo "Failed to save the file.";
}
}
?>
HTML Form
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Upload File from URL</title>
</head>
<body>
<form action="upload.php" method="POST">
<label for="file_url">Enter File URL:</label>
<input type="url" name="file_url" id="file_url" required>
<button type="submit">Upload</button>
</form>
</body>
</html>
2. Handling Redirects and HTTP Errors
Sometimes, the target URL might redirect or result in an HTTP error. Here’s how to handle these cases:
- Use cURL for Better Control
cURL provides detailed control over HTTP requests, allowing you to manage redirects and capture response codes.
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true); // Follow redirects
$fileContents = curl_exec($ch);
$httpCode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
if ($httpCode !== 200) {
die("Failed to fetch the file. HTTP Status Code: $httpCode");
}
3. Managing Authorization for Restricted URLs
If the URL requires authentication (e.g., API keys, HTTP Basic Auth), you can pass these credentials with cURL:
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($ch, CURLOPT_HTTPHEADER, [
'Authorization: Bearer YOUR_ACCESS_TOKEN'
]);
$fileContents = curl_exec($ch);
curl_close($ch);
if ($fileContents === false) {
die("Failed to fetch the file with authorization.");
}
4. Security Considerations
- Validate the URL: Ensure it’s a valid URL and not pointing to a malicious or unexpected location.
- Limit File Types: Check the MIME type to allow only specific file formats.
$finfo = new finfo(FILEINFO_MIME_TYPE); $mimeType = $finfo->buffer($fileContents); $allowedMimeTypes = ['image/jpeg', 'image/png', 'application/pdf']; if (!in_array($mimeType, $allowedMimeTypes)) { die("Unsupported file type."); }
- Set a Timeout: Prevent long-running requests with cURL timeouts:
curl_setopt($ch, CURLOPT_TIMEOUT, 10);
Conclusion
Using PHP to upload files directly from a URL streamlines workflows and adds versatility to your web applications. By implementing error handling, managing redirects, and supporting authentication, you can create a robust file upload system that handles external URLs gracefully.