How to Return a 404 Header in PHP: Best Practices and Useful Tips
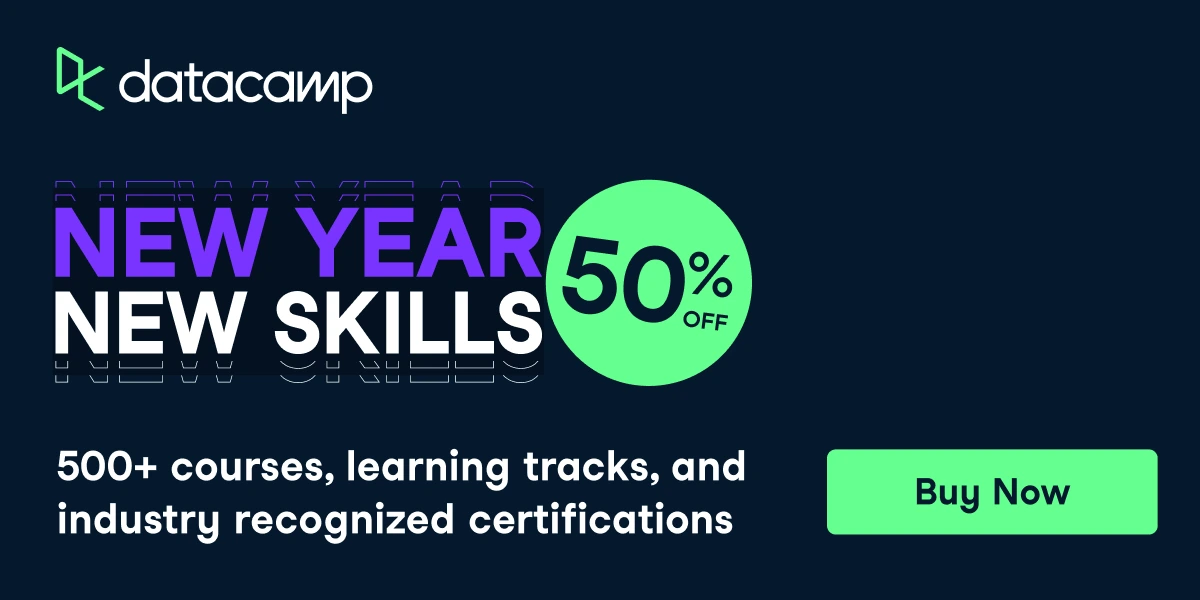
* Web 2.0 University is supported by it's audience. If you purchase through links on our site, we may earn an affiliate commision.
In web development, handling errors properly is critical to ensuring a smooth user experience and maintaining a well-functioning site.
One common task is returning a 404 error when a page is not found. The “404 Not Found” status tells browsers, search engines, and users that the requested resource doesn’t exist.
In PHP, generating a 404 response involves sending the appropriate HTTP header, and optionally, displaying a custom 404 error page.
In this blog post, we’ll dive into how to return a 404 header in PHP, what should be included in the response, and how libraries and frameworks make this easier.
How to Return a 404 Header from PHP
Returning a 404 status code from PHP is straightforward. You use the header()
function to send an HTTP status code before any other content or output is sent to the browser. Here’s the basic syntax for sending a 404 response:
<?php
header("HTTP/1.1 404 Not Found");
exit();
?>
In this example, the header()
function sends the HTTP 404 status to the client, and exit()
ensures that no further PHP code is executed.
Key Points to Remember:
- The
header()
function must be called before any output (e.g., HTML, echo statements, or whitespace) is sent to the browser. exit()
ordie()
is used to halt script execution after the header is sent.
What Should Be in the 404 Response?
When returning a 404 response, it’s important not only to send the appropriate header but also to provide the user with helpful content. A well-designed 404 page serves two purposes: informing the user that the requested page does not exist and offering navigational assistance to keep them engaged on your site.
Here are the key elements of a good 404 response:
1. The HTTP 404 Header
As mentioned, the most crucial part is sending the correct 404 header to inform the browser and search engines that the page is not found.
2. A User-Friendly 404 Page
You should design a custom 404 page that:
- Clearly states that the page could not be found.
- Provides a way back to your homepage or other relevant sections of your site.
- Optionally, includes a search box or links to popular content.
Here’s an example of returning both a 404 header and a custom 404 error page:
<?php
header("HTTP/1.1 404 Not Found");
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>404 - Page Not Found</title>
</head>
<body>
<h1>404 - Page Not Found</h1>
<p>Sorry, the page you are looking for does not exist. Please <a href="/">go back to the homepage</a>.</p>
</body>
</html>
3. SEO Considerations
If a page genuinely does not exist, search engines should be informed via the 404 status code so they don’t index the broken URL. Avoid returning a 200 OK status along with a custom 404 page, as this may cause search engines to think the page is valid.
Can a Header and a 404 Page Be Sent Back Together?
Yes, you can send both a 404 header and a custom HTML page simultaneously. This is a common practice and works as demonstrated in the example above. The browser receives the 404 HTTP status code, while users are presented with a user-friendly error page, ensuring that search engines and users alike get the proper feedback.
Here’s a breakdown of how this works:
- Header: Tells the client and search engines that the resource was not found (HTTP/1.1 404 Not Found).
- Body (HTML page): Shows a customized message to the user, helping them navigate back to your site’s valid pages.
By combining both, you provide a great user experience without compromising SEO.
How Libraries and Frameworks Help with 404 Errors
Many modern PHP frameworks and libraries have built-in support for handling 404 errors, which simplifies the process of managing different HTTP statuses and creating custom error pages.
1. Laravel
In Laravel, 404 errors are automatically handled. The framework catches missing routes or non-existent resources and returns a default 404 response. You can easily customize the 404 page by modifying the resources/views/errors/404.blade.php
file.
Example:
// In routes/web.php
Route::fallback(function () {
return response()->view('errors.404', [], 404);
});
This fallback()
route ensures that any invalid URL triggers a 404 response, along with the custom 404 Blade view.
2. Symfony
Symfony provides a similar mechanism for handling 404 errors. The framework automatically catches these errors and uses the Twig templating engine to display a custom error page.
To customize the 404 error page, create a template like templates/bundles/TwigBundle/Exception/error404.html.twig
. Symfony will handle the 404 header and render your page.
3. Slim Framework
Slim is a lightweight PHP framework that also simplifies handling 404 errors. You can define a custom 404 handler as shown below:
// In your Slim app configuration
$app->setNotFoundHandler(function ($request, $response) {
return $response
->withStatus(404)
->withHeader('Content-Type', 'text/html')
->write('Custom 404 Page - Not Found');
});
Slim automatically sends the 404 status and renders your custom content.
4. CakePHP
In CakePHP, 404 errors are handled via the ErrorController
. You can customize the error pages by modifying the src/Template/Error/error400.ctp
file for 400-level errors.
Conclusion
Handling 404 errors is a vital part of web development, as it ensures that users and search engines receive the proper feedback when pages don’t exist. In PHP, returning a 404 header is as simple as using the header()
function, but to enhance user experience, it’s best to include a custom error page with helpful navigation options.
Frameworks like Laravel, Symfony, Slim, and CakePHP make error handling even easier by providing built-in mechanisms to manage 404 responses and customize error pages. Following these best practices helps you create a better user experience, prevent broken links from harming your SEO, and maintain a cleaner web application.