How to Create a QR Code Generator with PHP (including logo)
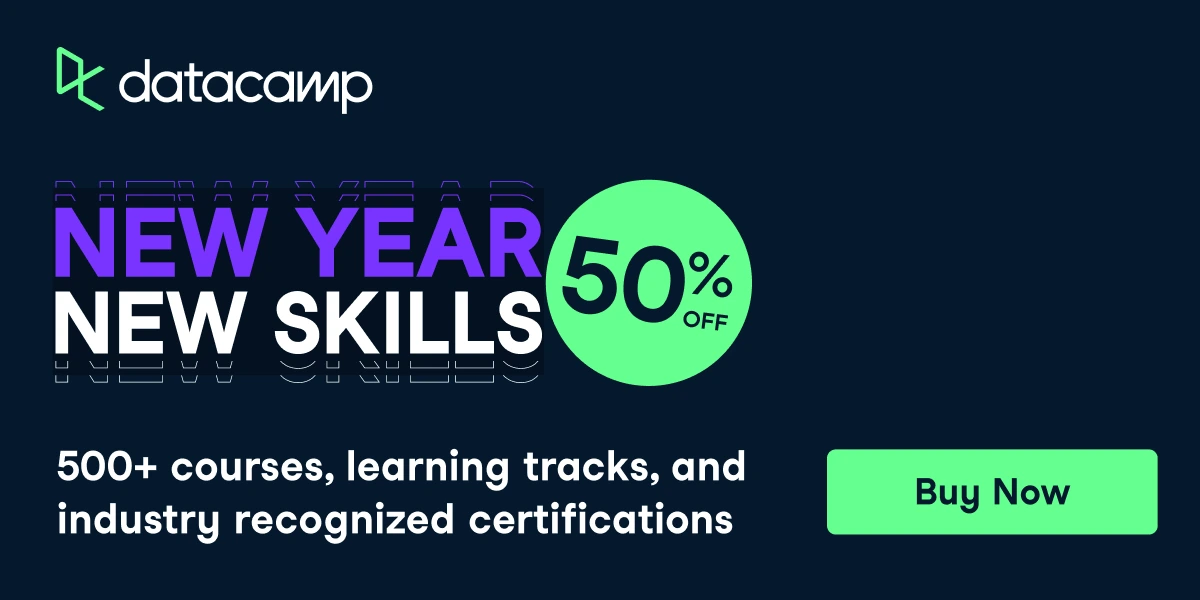
* Web 2.0 University is supported by it's audience. If you purchase through links on our site, we may earn an affiliate commision.
QR codes have become ubiquitous in our daily lives, offering a quick and convenient way to share information. Whether it’s for marketing, authentication, or simple data sharing, QR codes are an essential tool. But plain QR codes can sometimes be bland. Adding a logo to your QR code not only enhances its aesthetic appeal but also strengthens brand recognition. In this blog post, we’ll explore how to create a QR code with a logo using PHP.
Prerequisites
To generate QR codes with logos in PHP, you’ll need the following:
- PHP environment: Ensure you have PHP installed on your server or local machine.
- Composer: The dependency manager for PHP.
- Libraries: We’ll use the
endroid/qr-code
library for generating QR codes andintervention/image
for image manipulation.
Setting Up the Environment
First, set up your PHP environment and install the necessary libraries using Composer. Open your terminal and navigate to your project directory. Run the following command:
composer require endroid/qr-code
composer require intervention/image
Generating a Basic QR Code
Let’s start by generating a basic QR code. Create a PHP file, for example, generate_qr.php
, and add the following code:
<?php
require 'vendor/autoload.php';
use Endroid\QrCode\QrCode;
use Endroid\QrCode\Writer\PngWriter;
// Create a basic QR code
$qrCode = new QrCode('https://yourwebsite.com');
$qrCode->setSize(300);
// Create a writer and generate the QR code
$writer = new PngWriter();
$result = $writer->write($qrCode);
// Save the QR code to a file
$result->saveToFile(__DIR__ . '/basic_qr.png');
echo "QR code generated successfully!";
?>
This script generates a basic QR code pointing to https://yourwebsite.com
and saves it as basic_qr.png
in your project directory.
Adding a Logo to the QR Code
To add a logo to the QR code, we’ll use the intervention/image
library. Modify the generate_qr.php
file to include the logo:
<?php
require 'vendor/autoload.php';
use Endroid\QrCode\QrCode;
use Endroid\QrCode\Writer\PngWriter;
use Intervention\Image\ImageManagerStatic as Image;
// Create a basic QR code
$qrCode = new QrCode('https://yourwebsite.com');
$qrCode->setSize(300);
// Create a writer and generate the QR code
$writer = new PngWriter();
$result = $writer->write($qrCode);
// Load the QR code image
$qrImage = Image::make($result->getDataUri());
// Load the logo
$logo = Image::make('path/to/your/logo.png')->resize(70, 70);
// Calculate the position of the logo
$qrSize = $qrCode->getSize();
$logoPosX = ($qrSize / 2) - ($logo->width() / 2);
$logoPosY = ($qrSize / 2) - ($logo->height() / 2);
// Insert the logo into the QR code
$qrImage->insert($logo, 'top-left', (int)$logoPosX, (int)$logoPosY);
// Save the final QR code with logo
$qrImage->save(__DIR__ . '/qr_with_logo.png');
echo "QR code with logo generated successfully!";
?>
In this script, the logo image is loaded and resized to fit within the QR code. The logo is then positioned at the center of the QR code, and the combined image is saved as qr_with_logo.png
.
Practical Examples
Example 1: Generating a QR Code for a Website
If you want to generate a QR code for a website URL, simply change the URL in the $qrCode
object:
$qrCode = new QrCode('https://example.com');
Example 2: Generating a QR Code for Contact Information
You can also generate a QR code for contact information (vCard):
$vcard = "BEGIN:VCARD\n";
$vcard .= "VERSION:3.0\n";
$vcard .= "FN:John Doe\n";
$vcard .= "ORG:Company Name\n";
$vcard .= "TEL:+1234567890\n";
$vcard .= "EMAIL:[email protected]\n";
$vcard .= "END:VCARD";
$qrCode = new QrCode($vcard);
Conclusion
Creating QR codes with logos in PHP is a straightforward process thanks to powerful libraries like endroid/qr-code
and intervention/image
. By following the steps outlined in this guide, you can generate custom QR codes that not only serve their functional purpose but also enhance your brand’s visibility and appeal. Whether you’re sharing website URLs, contact information, or any other data, adding a logo to your QR code makes it more professional and engaging.