Php Remove Quotes From String
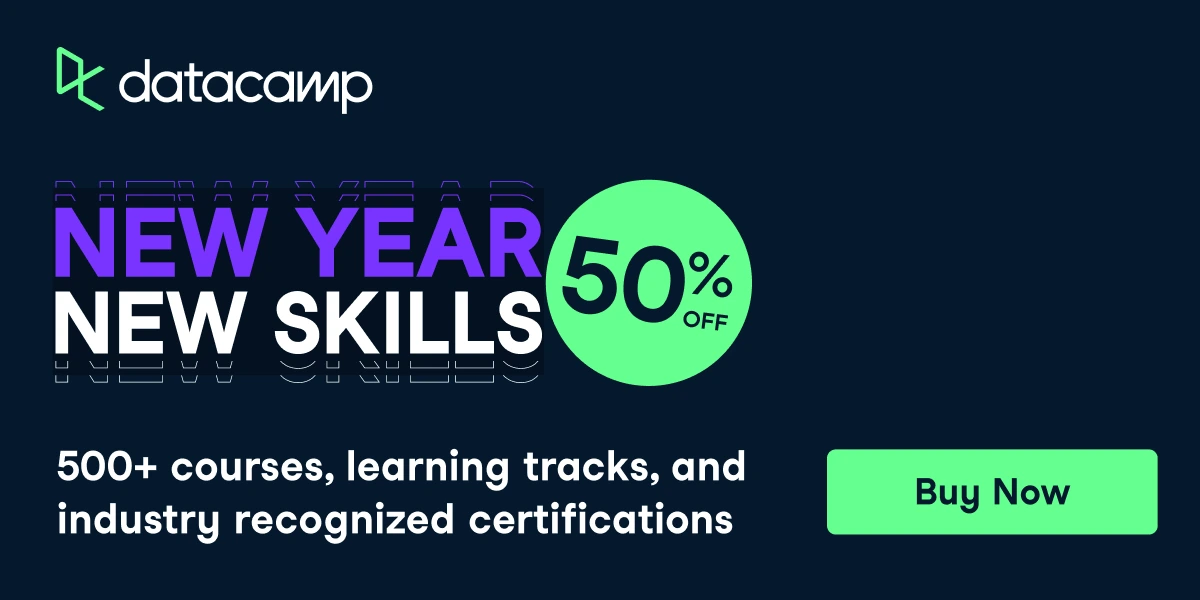
* Web 2.0 University is supported by it's audience. If you purchase through links on our site, we may earn an affiliate commision.
How to Remove Quotes from a String in PHP
Quotes are an essential part of working with strings in PHP, but there are times when you might need to remove them for cleaner data processing or formatting. Whether it’s single quotes, double quotes, or both, PHP provides several methods to strip these characters from strings effectively. In this guide, we’ll explore multiple approaches to achieve this, covering various scenarios and use cases.
Introduction
When dealing with input strings in PHP, you may encounter cases where quotes—either single ('
) or double ("
)—need to be removed. This can be especially useful when processing user inputs, cleaning up data, or preparing strings for further manipulation. In this post, we’ll demonstrate how to remove quotes from strings in PHP, comparing different methods and their applications.
Methods to Remove Quotes from Strings in PHP
1. Using str_replace
to Remove Quotes
The str_replace
function allows you to replace occurrences of specific characters in a string. To remove quotes, you simply replace them with an empty string.
<?php
$string = '"Hello, World!"';
$result = str_replace(['"', "'"], '', $string);
echo $result; // Outputs: Hello, World!
?>
- Pros:
- Simple and straightforward.
- Can handle both single and double quotes simultaneously.
- Cons:
- Requires specifying all quote characters to remove.
2. Using trim
to Remove Quotes from Beginning and End
If you need to remove quotes only from the start and end of a string, trim
is an efficient choice.
<?php
$string = '"Hello, World!"';
$result = trim($string, '"'' );
echo $result; // Outputs: Hello, World!
?>
- Pros:
- Perfect for removing quotes that surround a string.
- Highly efficient for this specific use case.
- Cons:
- Doesn’t remove quotes inside the string.
3. Using Regular Expressions with preg_replace
Regular expressions provide a powerful way to strip quotes from strings, whether they appear at the beginning, end, or anywhere else.
<?php
$string = '"Hello, 'World!'"';
$result = preg_replace('/["']/', '', $string);
echo $result; // Outputs: Hello, World!
?>
- Pros:
- Flexible and customizable.
- Handles complex patterns beyond just quotes.
- Cons:
- Slightly more complex syntax.
4. Using stripslashes
for Escaped Quotes
If your string contains escaped quotes (e.g., \"
), you can use stripslashes
to remove the backslashes.
<?php
$string = '\"Hello, World!\"';
$result = stripslashes($string);
echo $result; // Outputs: "Hello, World!"
?>
- Pros:
- Ideal for cleaning up strings with escape sequences.
- Cons:
- Does not remove the quotes themselves.
5. Combining stripslashes
and str_replace
For strings with escaped quotes that need to be removed entirely, combine stripslashes
and str_replace
.
<?php
$string = '\"Hello, World!\"';
$cleaned = str_replace('"', '', stripslashes($string));
echo $cleaned; // Outputs: Hello, World!
?>
Use Cases
Remove Double Quotes from Start and End To specifically remove double quotes wrapping a string:
$result = trim($string, '"');
Remove Single Quotes in a String To target single quotes within a string:
$result = str_replace("'", '', $string);
Ignore Quotes for Processing If quotes don’t need to be stripped but ignored for a specific operation, consider using regular expressions or parsing tools.
Comparison of Methods
Method | Best For | Complexity | Efficiency |
---|---|---|---|
str_replace | General removal of quotes | Low | High |
trim | Removing quotes from start and end | Low | High |
preg_replace | Complex patterns or all occurrences | Medium | Medium |
stripslashes | Escaped quotes | Low | Medium |
Combination (e.g., stripslashes + str_replace ) | Cleaning complex strings | Medium | Medium |
Conclusion
Removing quotes from a string in PHP can be achieved using various methods, depending on the specific requirements. For simple tasks, functions like str_replace
and trim
are highly effective, while preg_replace
offers more flexibility for complex scenarios. Understanding these methods ensures you’re prepared to handle any quote-removal challenge in your PHP projects.