Getting Started with PHPMailer: A Simple Guide to Sending Emails with PHP
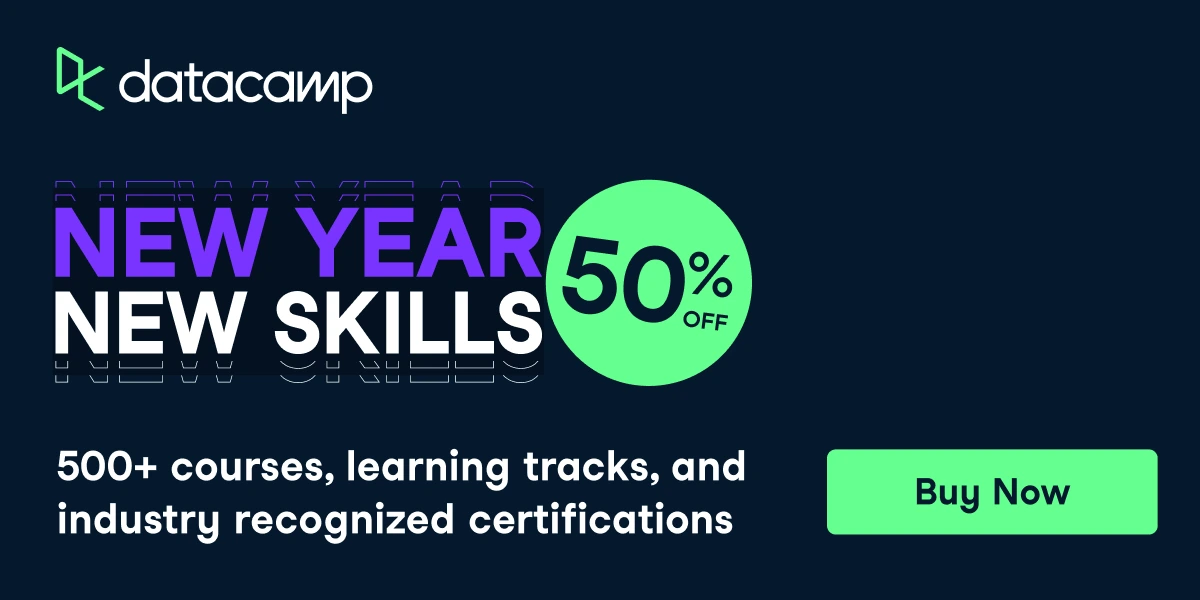
* Web 2.0 University is supported by it's audience. If you purchase through links on our site, we may earn an affiliate commision.
Sending emails is a crucial feature in web development, whether it’s for contact forms, newsletters, or user notifications. While PHP has a built-in mail()
function, many developers find it limited in terms of functionality and security. This is where PHPMailer comes in—a widely-used and highly customizable library for sending emails via PHP. It provides better error handling, supports attachments, and integrates easily with third-party email services like Gmail and SMTP.
In this post, we will explore what PHPMailer is, how to send a simple email, whether it works on shared hosting, and provide a full example of sending an email through a web form.
What is PHPMailer?
PHPMailer is an open-source PHP library for sending emails in a flexible and secure way. It offers many features that the built-in mail()
function lacks, including:
- Sending emails via SMTP, which is more reliable than the PHP
mail()
function. - Better error handling, so you know when something goes wrong.
- Support for HTML content, attachments, and CC/BCC.
- Authentication support, including SMTP and OAuth.
PHPMailer has become the go-to library for email functionality in PHP applications due to its robust feature set and ease of use.
How to Send a Simple Mail with PHPMailer
To send a simple email using PHPMailer, follow these steps:
Step 1: Install PHPMailer
First, install PHPMailer using Composer, the recommended way to manage dependencies in PHP.
composer require phpmailer/phpmailer
Alternatively, you can manually download the PHPMailer package and include the necessary files in your project.
Step 2: Basic Email Script with PHPMailer
Here is a basic script for sending an email using PHPMailer:
<?php
// Import PHPMailer classes
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
// Load Composer's autoloader or manually include required files
require 'vendor/autoload.php';
$mail = new PHPMailer(true);
try {
//Server settings
$mail->isSMTP(); // Use SMTP
$mail->Host = 'smtp.example.com'; // Set the SMTP server to send through
$mail->SMTPAuth = true; // Enable SMTP authentication
$mail->Username = '[email protected]'; // SMTP username
$mail->Password = 'your_email_password'; // SMTP password
$mail->SMTPSecure = 'tls'; // Enable TLS encryption; `ssl` also accepted
$mail->Port = 587; // TCP port to connect to
//Recipients
$mail->setFrom('[email protected]', 'Mailer');
$mail->addAddress('[email protected]', 'Recipient Name'); // Add a recipient
$mail->addReplyTo('[email protected]', 'Information');
// Content
$mail->isHTML(true); // Set email format to HTML
$mail->Subject = 'Here is the subject';
$mail->Body = 'This is the HTML message body <b>in bold!</b>';
$mail->AltBody = 'This is the body in plain text for non-HTML mail clients';
$mail->send();
echo 'Message has been sent';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
?>
In this example:
- You configure the SMTP settings, including the host, port, and authentication credentials.
- You set the sender and recipient email addresses.
- The email content is defined with both an HTML and a plain-text alternative for non-HTML clients.
Can PHPMailer Be Used on Shared Hosting like HostGator?
Yes, PHPMailer can be used on shared hosting environments like HostGator. Shared hosting services typically support PHP and allow you to send emails either using an external SMTP server or the hosting provider’s own SMTP service.
If you’re using shared hosting:
- Make sure the SMTP ports (such as 587 or 465) are not blocked by your host. Many shared hosts allow SMTP for sending emails, but it’s always best to check with your provider.
- You may need to configure PHPMailer to use the SMTP server provided by your hosting company or third-party services like Gmail.
Here’s an example configuration for Gmail SMTP on shared hosting:
$mail->isSMTP();
$mail->Host = 'smtp.gmail.com';
$mail->SMTPAuth = true;
$mail->Username = '[email protected]';
$mail->Password = 'your_gmail_password';
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
Make sure to use an App Password for Gmail, as Google now requires app-specific passwords for non-Google apps (like PHPMailer).
Full Web Form Example Sending a Mail Using PHPMailer
Let’s build a simple web form that collects a user’s name, email, and message, and then sends it using PHPMailer.
Step 1: Create an HTML Form
<!DOCTYPE html>
<html>
<head>
<title>Contact Form</title>
</head>
<body>
<h2>Contact Us</h2>
<form action="send_mail.php" method="POST">
<label for="name">Name:</label>
<input type="text" name="name" required><br><br>
<label for="email">Email:</label>
<input type="email" name="email" required><br><br>
<label for="message">Message:</label>
<textarea name="message" required></textarea><br><br>
<input type="submit" value="Send Message">
</form>
</body>
</html>
Step 2: Handle the Form Submission and Send the Email
In your send_mail.php
file:
<?php
use PHPMailer\PHPMailer\PHPMailer;
use PHPMailer\PHPMailer\Exception;
require 'vendor/autoload.php'; // Use Composer's autoload
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$name = htmlspecialchars($_POST['name']);
$email = htmlspecialchars($_POST['email']);
$message = htmlspecialchars($_POST['message']);
$mail = new PHPMailer(true);
try {
//Server settings
$mail->isSMTP();
$mail->Host = 'smtp.example.com';
$mail->SMTPAuth = true;
$mail->Username = '[email protected]';
$mail->Password = 'your_email_password';
$mail->SMTPSecure = 'tls';
$mail->Port = 587;
//Recipients
$mail->setFrom($email, $name);
$mail->addAddress('[email protected]', 'Website Admin');
// Content
$mail->isHTML(true);
$mail->Subject = 'New Message from Contact Form';
$mail->Body = "Name: $name<br>Email: $email<br>Message: $message";
$mail->AltBody = "Name: $name\nEmail: $email\nMessage: $message";
$mail->send();
echo 'Message has been sent successfully.';
} catch (Exception $e) {
echo "Message could not be sent. Mailer Error: {$mail->ErrorInfo}";
}
}
?>
In this form:
- User inputs (name, email, message) are passed to
send_mail.php
via POST. - PHPMailer sends the email using SMTP settings, with the sender details included.
Conclusion
PHPMailer is an incredibly useful library for handling email sending in PHP. It offers more flexibility, security, and error handling than the default PHP mail()
function. Whether you’re on a dedicated server or shared hosting like HostGator, PHPMailer can handle your email needs via SMTP, with easy integration for services like Gmail.
In this post, we covered the basics of PHPMailer, from sending a simple email to integrating it with a web form. With libraries like PHPMailer, sending robust and secure emails becomes a much more manageable task for developers.